I’m excited to try out the new Claude API from Anthropic! This API allows you to access Claude, Anthropic’s powerful conversational AI assistant, directly from your Python code.
Getting started with the Claude API is easy. First, you’ll need to sign up and get an API key. Once you have your key, you can start passing prompts to Claude and streaming back responses. Here’s a simple example, assuming you have exported your API key using: export ANTROPHIC_API_KEY=KEY
import anthropic client = anthropic.Anthropic( api_key="ANTHROPIC_API_KEY" # or export ANTROPHIC_API_KEY=KEY ) message = client.messages.create( model="claude-2.1", max_tokens=1024, messages=[ {"role": "user", "content": "Give a 4 day itinerary to Rio de Janeiro in Brazil for our family."} ] ) print(message.content[0].text)
And if you want to have a streaming response:
import anthropic client = anthropic.Anthropic() with client.messages.stream( model="claude-2.1", max_tokens=1024, messages=[ {"role": "user", "content": "Give a 3 day itinerary to Tokyo, Japan."} ] ) as stream: for text in stream.text_stream: print(text, end="", flush=True)
Here is a 3 day suggested itinerary for visiting Tokyo, Japan:
Day 1:
– Meiji Jingu Shrine – Visit this peaceful, forested shrine in the morning.
– Harajuku – Shop and people watch in the popular and trendy Harajuku district. Get lunch at one of the themed cafes.
– Shibuya Crossing – See the famous Shibuya scramble intersection and Shibuya 109 shopping area.
– Shinjuku Gyoen Park – Walk through the beautiful gardens for a nice afternoon break.
– Golden Gai – Explore the tiny alleyways full of tiny bars and eateries. Have dinner at an izakaya.
Day 2:
– Tokyo Fish Market – Go early to the world-famous fish and seafood market. Have sushi for breakfast.
– Senso-ji Temple – See the iconic temple in Asakusa with markets and shops.
– Tokyo Skytree – Go up Tokyo’s tallest structure for amazing views. Shop and eat lunch in the Solamachi complex at the base.
– Akihabara – Spend the afternoon in the anime and video game capital of the world. Play arcade games, shop for manga souvenirs.
– Izakaya Dinner – Eat and drink in a casual Japanese tavern.
Day 3:
– Tokyo National Museum – See fine art, sculpture, calligraphy and archeology exhibits in the morning.
– Imperial Palace East Garden – Take a free tour of the lovely gardens on the palace grounds.
– Ginza – Walk along the main street of this elegant upscale shopping district. Have a nice lunch or tea here.
– Tokyo Tower – Get scenic views of the city from this iconic red tower.
– Odaiba – Cross the rainbow bridge to this entertainment district. Visit museums like Miraikan, go shopping, play in arcades. Have a nice seafood dinner.
Now lets add a quick UI to interact with the model with help from Gradio:
import anthropic import gradio as gr def query_anthropic_model(user_question): client = anthropic.Anthropic() message = client.messages.create( model="claude-2.1", max_tokens=1024, messages=[ {"role": "user", "content": user_question} ] ) return message.content[0].text iface = gr.Interface(fn=query_anthropic_model, inputs=gr.Textbox(lines=2, placeholder="Enter your question here..."), outputs="text", title="Anthropic Model Query Interface", description="Type your question to get an answer from the Anthropics model." ) iface.launch()
and the final result:
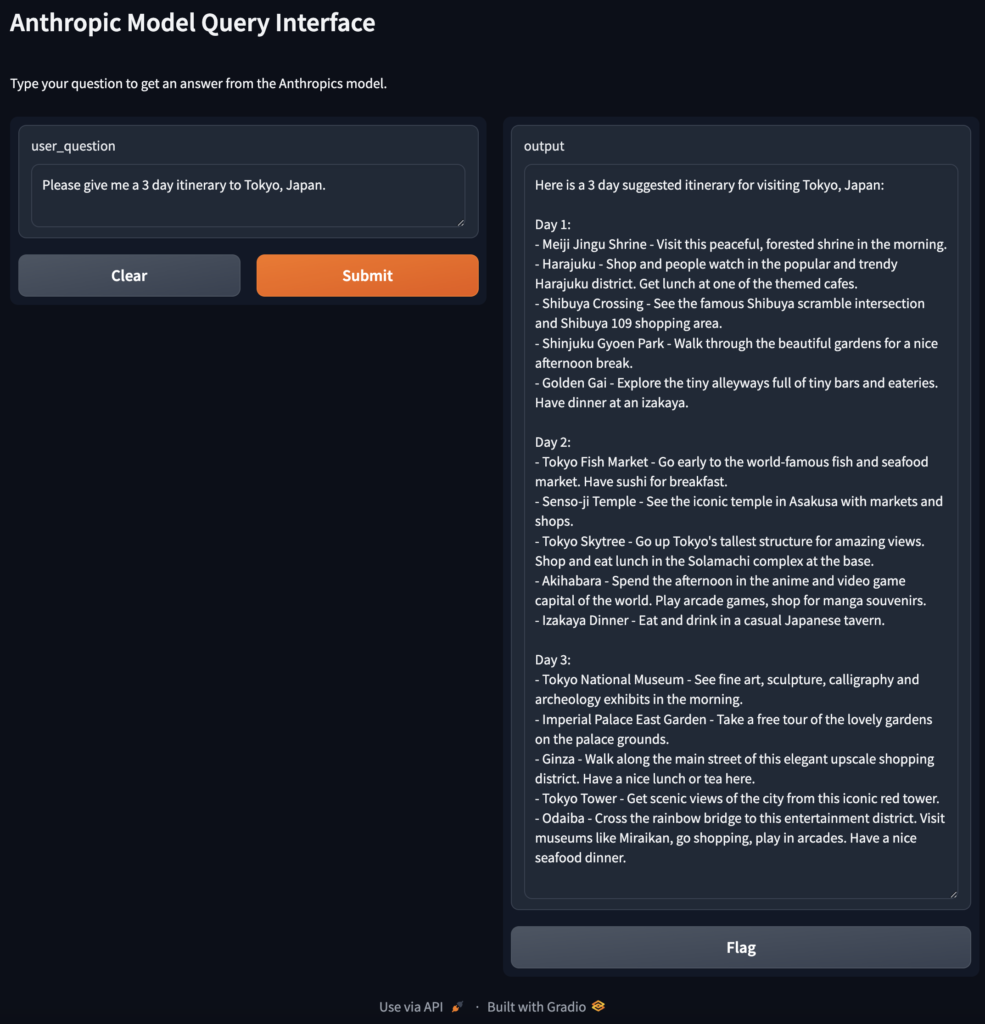